第一节 在IOC容器中装配Bean
1.1Spring容器成功启动条件
1.导入Spring框架相关的jar包
2.正确配置spring配置文件
3.Bean的类都已放到应用程序的类路径下
1.2Bean配置信息的组成
Bean配置信息是Bean的元数据信息(类的信息)
1.Bean的实现类
2.Bean的属性信息
3.Bean的依赖信息
4.Bean的行为配置
1.3xml、bean、容器、应用程序之间的关系!
1.4基于xml的配置
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.0.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-4.3.xsd">
<bean id="phone" class="com.test.testDom4j.Phone">
<property name="brand" value="小米"></property>
<property name="type" value="6s"></property>
<property name="price" value="2500"></property>
</bean>
<bean id="car" class="com.test.testSpring.Car">
<constructor-arg value="1" index="0"></constructor-arg>
<constructor-arg value="宝马" index="1"></constructor-arg>
<constructor-arg value="95.6" index="2"></constructor-arg>
</bean>
<bean id="car1" class="com.test.testSpring.Car">
<constructor-arg value="2" index="0"></constructor-arg>
<constructor-arg value="奥迪" index="1"></constructor-arg>
<constructor-arg value="65" index="2"></constructor-arg>
</bean>
<!-- 给list集合赋值 -->
<bean id="person" class="com.test.testSpring.Person">
<property name="name">
<value>沈志龙</value>
</property>
<property name="age" >
<value>26</value>
</property>
<property name="cars">
<list>
<ref bean="car"/>
<ref bean="car1"/>
</list>
</property>
</bean>
<!-- 给map集合赋值 -->
<bean id="person1" class="com.test.testSpring.Person2">
<property name="name">
<value>沈志龙</value>
</property>
<property name="age" >
<value>26</value>
</property>
<property name="cars">
<map>
<entry key="baoma" value-ref="car"></entry>
<entry key="aodi" value-ref="car1"></entry>
</map>
</property>
</bean>
<!-- 配置propertites -->
<bean id="p1" class="com.test.testSpring.DataSource">
<property name="properties">
<props>
<prop key="url">jdbc:mysql:///jb</prop>
<prop key="driver">com.mysql.jdbc.Driver</prop>
<prop key="username">root</prop>
<prop key="username">root</prop>
<prop key="password">as501226107A.</prop>
</props>
</property>
</bean>
<!-- 配置公共的list -->
<util:list id="cars">
<ref bean="car"/>
<ref bean="car1"/>
</util:list>
<bean id="person2" class="com.test.testSpring.Person">
<property name="name" value="刘德华"></property>
<property name="age" value="57"></property>
<property name="cars" ref="cars"></property>
</bean>
</beans>
第二节 Bean基本配置
2.1.1装配一个bean
Spring配置文件中定义一个简要的bean配置片段
<bean id="Foo" class="com.dream.Foo"></bean>
2.1.2Bean的命名
在配置bean的时候,需要为bean指明一个id作为bean的名称,id在IOC容器中是唯一的。
name属性
可以使用特殊字符. 也可以根据name属性获取一个对象!
id属性
id属性作用和name几乎相同,但是也有细微的差别,id不可重复,且不能使用特殊字符
的引用
2.1.3使用 p 命名空间
• 为了简化 XML 文件的配置,越来越多的 XML 文件采用属性而非子元素配置信息。
• Spring 从 2.5 版本开始引入了一个新的 p 命名空间,可以通过 元素属性的方式配置 Bean 的属性。
• 使用 p 命名空间后,基于 XML 的配置方式将进一步简化• 为了简化 XML 文件的配置,越来越多的 XML 文件采用属性而非子元素配置信息。
• Spring 从 2.5 版本开始引入了一个新的 p 命名空间,可以通过 元素属性的方式配置 Bean 的属性。
• 使用 p 命名空间后,基于 XML 的配置方式将进一步简化
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd">
<bean name="john-classic" class="com.example.Person">
<property name="name" value="John Doe"/>
<property name="spouse" ref="jane"/>
</bean>
<bean name="john-modern"
class="com.example.Person"
p:name="John Doe"
p:spouse-ref="jane"/>
<bean name="jane" class="com.example.Person">
<property name="name" value="Jane Doe"/>
</bean>
</beans>
上面的例子不仅使用p名称空间包含了一个属性(property)值,而且使用了一个特殊的格式声明了一个属性引用。在第一个bean定义中使用了`<property name="spouse" ref="jane"/>`来建立bean`john`到bean`jane`的引用,而第二个bean定义则采用`p:spouse-ref="jane"`属性(attribute)的方式达到了同样的目的。在这个例子中,"`spouse`"是属性(property)名,而"`-ref`“则用来说明该属性不是一个具体的值而是对另外一个bean的引用。
2.1.4lazy-init属性
注意: 只对单例有效,设置**scope=“singleton”**时测试
延时创建属性.
lazy-init=“false” 默认值,不延迟创建,即在框架启动时候就创建对象.
lazy-init=“true” **延迟初始化,**在用到对象的时候才会创建对象.
配置:
第三节 依赖注入
3.0理解IOC和DI
控制反转:之前对象的控制权在我们手上,对象的创建、销毁都需要我们来控制,但是现在,SpringIoc容器可以帮我们去完成这一系列事情,当我们需要用到某个对象的时候,我们只需要告诉容器,容器会为我们找到并提供合适的对象,而我们只需要去关注如何使用
依赖注入:容器为其对象注入依赖属性的过程
3.1属性注入
3.1.1 Spring属性注入实例
1.属性注入是通过setXXX()方法注入Bean的属性值或者依赖对象
2.属性注入需要无参构造方法,容器需要通过反射来创建对象
<bean id="phone" class="com.test.testDom4j.Phone">
<property name="brand" value="小米"></property>
<property name="type" value="6s"></property>
<property name="price" value="2500"></property>
</bean>
3.1.2 参数及元素名介绍
id: bean的唯一名称,容器通过id来获取bean
class:该bean类的全路径,容器通过获取路径并使用反射的技术来创建实例
property: bean的属性配置
name:属性名称,对应类的属性
value:属性的值(value也可以写在property的内部)
<property name="name">
<value>沈志龙</value>
</property>
3.1.6
常规做法
public class UserDao{
public void addUser(String username) {
System.out.println("添加用户:"+username);
}
}
public class UserMessage {
String username="demo";
UserDao userDao;
public UserMessage(){
userDao=new UserDao();
}
public void addUser(){
userDao.addUser(username);
}
}
public class test{
UserMessage userMessage=new UserMessage();
userMessage.addUser();
}
由于在UserMessage内部创建了UserDao对象,导致两个类的耦合度较高,如果需要另一个Dao类来实现addUser方法,需要更改UserMessage的代码,重新new另一个类,如果系统中有很多地方用到这个Dao类,那么我们需要修改很多次,出现Bug的几率很高,所以不推荐这种做法
面向接口
public interface UserDao{
void addUser(String username);
}
public class UserDaoImpl implements UserDao{
@Override
public void addUser(String username)
{
System.out.println("添加用户:"+username);
}
}
public class UserMessage{
private UserDao userDao;
//使用设值方式赋值
public void setUserDao(UserDao userDao) {
this.userDao = userDao;
}
public void addUser(String userName, String password) {
userDao.addUser(userName);
}
}
public class test{
public static void main(String[] args) {
UserDao userDao =new UserDaoImpl();
UserMessage userMessage=new UserMessage();
userMessage.setUserDao(userDao);
}
}
使用面向接口的思想降低了两个类之间的耦合度,我们如果需要修改addUser的逻辑,我们只需要修改实现类中的代码,并不会去修改UserMessage的代码
Spring依赖注入
在面向接口的基础上来,通过依赖注入来将所依赖的对象注入到Bean中
userManager.xml
<!-- 使用spring管理对象的创建,还有对象的依赖关系 -->
<bean id="userManager" class="scau.zzf.service.UserMessage">
<!-- (1)UserMessageImpl使用了userDao,Ioc是自动创建相应的UserDao实现,都是由容器管理-->
<!-- (2)在UserMessageImpl中提供构造函数,让spring将UserDao实现注入(DI)过来 -->
<!-- (3)让spring管理我们对象的创建和依赖关系,必须将依赖关系配置到spring的核心配置文件中 -->
<property name="userDao" ref="UserDao"></property>
<!-- 构造注入 -->
<!-- <constructor-arg ref="userDao"/> -->
</bean>
<bean id="UserDao" class="scau.zzf.Dao.UserDao">
</bean>
public interface UserDao{
void addUser(String username);
}
public class UserDaoImpl implements UserDao{
@Override
public void addUser(String username)
{
System.out.println("添加用户:"+username);
}
}
public class UserMessage{
@Autowired
private UserDao userDao;
//使用设值方式赋值
public void setUserDao(UserDao userDao) {
this.userDao = userDao;
}
public void addUser(String userName, String password) {
userDao.addUser(userName);
}
}
public class test{
public static void main(String[] args) {
ApplicationContext atc=new ClassPathXmlApplicationContext("userManager.xml");
UserMessage userMessage=(UserMessage) atc.getBean("userMessage");
//使用 UserMessage userMessage=atc.getBean(UserMessage.class,"userMessage");不用强制转化
userMessage.addUser("xx","xx");
}
}
在创建容器的时候,容器会扫描xml中的bean配置信息,并实例化相应的Bean添加到Spring容器中,在用户需要使用Bean的时候,就可以通过传入ApplicationContext的实例对象的getBean方法,传入bean的id获取相应的实例。
3.2 构造函数注入
3.2.1 按类型匹配注入
public class Car {
private int id;
private String type;
private double price;
private String place;
/**
* @param id
* @param type
* @param price
*/
public Car(int id, String type, double price) {
this.id = id;
this.type = type;
this.price = price;
}
/**
*
*/
public Car() {
super();
// TODO Auto-generated constructor stub
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String getPlace() {
return place;
}
public void setPlace(String place) {
this.place = place;
}
@Override
public String toString() {
return "Car [id=" + id + ", type=" + type + ", price=" + price + ", place=" + place + "]";
}
}
<bean id="car" class="com.test.testSpring.Car">
<constructor-arg value="1" type="int"></constructor-arg>
<constructor-arg value="宝马" type="java.lang.String"></constructor-arg>
<constructor-arg value="95.6"></constructor-arg>
</bean>
注意
1.如果不设置参数类型,则按照默认的constructor-arg配置顺序去匹配,如果此时参数类型不对则会产生异常
警告: Exception encountered during context initialization - cancelling refresh attempt: org.springframework.beans.factory.UnsatisfiedDependencyException: Error creating bean with name 'car2' defined in class path resource [applicationContext.xml]: Unsatisfied dependency expressed through constructor parameter 0: Could not convert argument value of type [java.lang.String] to required type [int]: Failed to convert value of type 'java.lang.String' to required type 'int'; nested exception is java.lang.NumberFormatException: For input string: "奥迪"
2.可以代表构造方法的入参顺序吗?
如果只有一个相同入参类型的构造方法,可以代表;但是如果存在多个具有相同类型入参的构造方法,它的顺序标识就失效了
3.的顺序不会对最终的配置结果产生影响,但是如果存在多个相同入参类型的构造方法,则需要使用到索引匹配和类型匹配的配合
3.2.2按照索引匹配注入
public Car( String place,String type,double price) {
this.type = type;
this.price = price;
this.place = place;
}
此时如果使用类型注入,分不清第一个和第二个是到底是注入的哪个属性,产生了不确定性,此时就需要使 用索引匹配注入。
注意
1.index属性的下标从0开始,0代表第一个参数
2.通常联合使用类型和索引匹配入参
3.3 工厂方法注入
非静态工厂
创建工厂类
配置文件:applicationContext-create.xml
测试代码
静态工厂
.java
public class CustomerStaticFactory {
public static Customer getCustomer() {
System.out.println("静态工程创建了customer");
return new Customer();
}
}
context.xml
<!-- 静态工厂 -->
<bean id="customerStaticFactory" class="com.dream.bean.CustomerStaticFactory" factory-method="getCustomer"></bean>
测试
ApplicationContext context=new ClassPathXmlApplicationContext("bean1.xml");
Customer bean = (Customer) context.getBean("customerStaticFactory");
System.out.println(bean);
Customer bean1 = (Customer) context.getBean("customerStaticFactory");
System.out.println(bean1);
System.out.println(bean==bean1);
结果:
因为静态工厂是直接创建了一个新的对象,没有设置bean的singleton属性,默认是单例,所以对象只创建了一次,两次获得的是同一个对象,第一次创建了
将singleton设为prototype,
<bean id="customerStaticFactory" class="com.dream.bean.CustomerStaticFactory" factory-method="getCustomer" scope="prototype"></bean>
结果
第四节 注入参数详解
4.1.1 引用其它 Bean
• 组成应用程序的 Bean 经常需要相互协作以完成应用程序的功能. 要使 Bean 能够相互访问, 就必须在 Bean 配置文件中指定对 Bean 的引用
• 在 Bean 的配置文件中, 可以通过 元素或 ref 属性为 Bean 的属性或构造器参数指定对 Bean 的引用.
• 也可以在属性或构造器里包含 Bean 的声明, 这样的 Bean 称为内部 Bean
4.1.2 内部bean
• 当 Bean 实例仅仅给一个特定的属性使用时, 可以将其声明为内部 Bean. 内部 Bean 声明直接包含在 或 元素里, 不需要设置任何 id 或 name 属性
• 内部 Bean 不能使用在任何其他地方
4.1.3引入集合
Collection集合
• 在 Spring中可以通过一组内置的 xml 标签(例如: , 或
• 配置 java.util.List 类型的属性, 需要指定 标签, 在标签里包含一些元素. 这些标签可以通过 指定简单的常量值, 通过 指定对其他 Bean 的引用. 通过**** 指定内置 Bean 定义. 通过 指定空元素. 甚至可以内嵌其他集合.
• 数组的定义和 List 一样, 都使用
• 配置 java.util.Set 需要使用 标签, 定义元素的方法与 List 一样.
Map
• Java.util.Map 通过
• 必须在 标签里定义键
• 因为键和值的类型没有限制, 所以可以*地为它们指定 , , 或 元素.
• 可以将 Map 的键和值作为 的属性定义: 简单常量使用 key 和 value 来定义; Bean 引用通过 key-ref 和 value-ref 属性定义
• 使用 定义 java.util.Properties, 该标签使用多个 作为子标签. 每个 标签必须定义 key 属性.
4.1.4自动装配
• Spring IOC 容器可以自动装配 Bean. 需要做的仅仅是在 的 autowire 属性里指定自动装配的模式
• byType(根据类型自动装配): 若 IOC 容器中有多个与目标 Bean 类型一致的 Bean. 在这种情况下, Spring 将无法判定哪个 Bean 最合适该属性, 所以不能执行自动装配.
• byName(根据名称自动装配): 必须将目标 Bean 的名称和属性名设置的完全相同.
• constructor(通过构造器自动装配): 当 Bean 中存在多个构造器时, 此种自动装配方式将会很复杂. 不推荐使用
第五节 Bean 的作用域(5个)
• 在 Spring 中, 可以在 元素的 scope 属性里设置 Bean 的作用域.
• 默认情况下, Spring 只为每个在 IOC 容器里声明的 Bean 创建唯一一个实例, 整个 IOC 容器范围内都能共享该实例:所有后续的 getBean() 调用和 Bean 引用都将返回这个唯一的 Bean 实例.该作用域被称为 singleton, 它是所有 Bean 的默认作用域.s
gloabal session:
global session作用域类似于标准的HTTP Session作用域,不过它仅仅在基于portlet的web应用中才有意义。Portlet规范定义了全局Session的概念,它被所有构成某个portlet web应用的各种不同的portlet所共享。在global session作用域中定义的bean被限定于全局portlet Session的生命周期范围内。
第六节 使用外部属性文件
• 在配置文件里配置 Bean 时, 有时需要在 Bean 的配置里混入==系统部署的细节信息==(例如: 文件路径, 数据源配置信息等). 而这些部署细节实际上需要和 Bean 配置相分离
• Spring 提供了一个 PropertyPlaceholderConfigurer 的 BeanFactory 后置处理器, 这个处理器允许用户将 Bean 配置的部分内容外移到属性文件中. 可以在 Bean 配置文件里使用形式为 ${var} 的变量, PropertyPlaceholderConfigurer 从属性文件里加载属性, 并使用这些属性来替换变量.
• Spring 还允许在属性文件中使用 ${propName},以实现属性之间的相互引用。
<context:property-placeholder location=“classpath:db.properties”/>
第七节 整合多个配置文件
• Spring 允许通过 将多个配置文件引入到一个文件中,进行配置文件的集成。这样在启动 Spring 容器时,仅需要指定这个合并好的配置文件就可以。
• import 元素的 resource 属性支持 Spring 的标准的路径资源
第八节 基于注解的配置
8.1类头部可用的注解
使用@Compnent注解,该类可以被Spring容器识别,Spring容器将自动将标注的类转化为容器中的Bean
/*
* @Component(userDao) == <bean name="userDao" class="com.itqf.spring.bean.UserDao" />
*/
@Compnent("userDao")
public class UserDao{
....
}
等同于
<bean id="userDao" class="xx.xx.UserDao"/>
与@Compnent功能基本相同的三个注解:
@Repository :用于对DAO实现类进行标注
@Service:用于对Service实现类进行标注
@Controller:用于对Controller实现类进行标注
提供这三个注解是为了让标注类本身的用途清晰化
指定对象作用域
8.1.1扫描注解定义的Bean
Sping提供了一个context命名空间,它提供了通过扫描类包以应用Bean的方式
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd">
<context:component-scan base-package="com.test.annoation"></context:component-scan>
</beans>
Controller
/**
*
*/
package com.test.annoation;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.stereotype.Controller;
/**
* Class Name: BookController.java
* Description:
* @author dragon DateTime 2018年9月13日 下午8:14:20
* @company bvit
* @email [email protected]
* @version 1.0
*/
@Controller
public class BookController {
@Autowired
private BookService bookService;
public void bookAdd() {
System.out.println("BookController.bookAdd");
bookService.add();
}
}
Serivice
@Service
public class BookService {
@Autowired
private BookDao bookDao;
public void add() {
System.out.println("bookService .add");
bookDao.add();
}
}
Dao
public interface BookDao {
public void add();
}
@Repository
public class BookDaoImpl implements BookDao {
/* (non-Javadoc)
* @see com.test.annoation.BookDao#add()
*/
public void add() {
// TODO Auto-generated method stub
System.out.println("add");
}
}
对于扫描到的组件, **Spring** **有默认的命名策略**: 使用非限定类名, 第一个字母小写**.** **也可以在注解中通过 value** **属性值标识组件的名称**
• 当在组件类上使用了特定的注解之后, 还需要在 Spring 的配置文件中声明 context:component-scan
• base-package 属性指定一个需要扫描的基类包,Spring 容器将会扫描这个基类包里及其子包中的所有类.
• 当需要扫描多个包时, 可以使用逗号分隔.
8.2注入属性值的注解
1.设置成员变量上:通过反射给变量赋值
@Value(“name值”) 等同于 @Value(value=“name值”)
1.加在set方法上:通过set方法赋值
对类的过滤
• 如果仅希望扫描特定的类而非基包下的所有类,可使用 resource-pattern 属性过滤特定的类,示例:
• context:include-filter 子节点表示要包含的目标类
• context:exclude-filter 子节点表示要排除在外的目标类
• context:component-scan 下可以拥有若干个 context:include-filter 和 context:exclude-filter 子节点
过滤表达式
注意
注意
注意
context:component-scan 中拥有一个use-default-filters属性,其默认值为true,标识会默认扫描上述四个注解标注的Bean。如果想仅扫描@Controller中的bean,需要将该属性设置为false
8.1.3 自动装配Bean
• context:component-scan 元素还会自动注册 AutowiredAnnotationBeanPostProcessor 实例, 该实例可以自动装配具有 @Autowired 和 @Resource 、@Inject注解的属性.
使用 @Autowired 自动装配 Bean
@Autowired 注解自动装配具有兼容类型的单个 Bean属性
– 构造器, **普通字段(**即使是非 public), 一切具有参数的方法都可以应用@Authwired 注解
@Autowired会将方法中的参数Bean自动注入到方法中
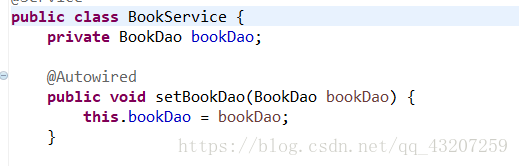
– 默认情况下, 所有使用 @Authwired 注解的属性都需要被设置. 当 Spring 找不到匹配的 Bean 装配属性时, 会抛出异常, 若某一属性允许不被设置, 可以设置 @Authwired 注解的 required 属性为 false
– 默认情况下, 当 IOC 容器里存在多个类型兼容的 Bean 时, 通过类型的自动装配将无法工作. 此时可以在 @Qualifier 注解里提供 Bean 的名称. Spring 允许对方法的入参标注 @Qualifiter 已指定注入 Bean 的名称
Person.java
@Component
public class Person{
@Value("龙哥")
private String name;
@Autowired
private Car car;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Car getCar() {
return car;
}
public void setCar(Car car) {
this.car = car;
}
}
接口即实现类:
//将Car定义成接口
interface Car {
void log();
}
//Baoma实现Car
@Component
class Baoma implements Car {
public void log() {
System.out.println("宝马");
}
}
//XianDai实现Car
@Component
class XianDai implements Car {
public void log() {
System.out.println("现代");
}
}
配置applicationContext.xml配置文件扫描包
测试
ApplicationContext context=new ClassPathXmlApplicationContext("bean1.xml");
Person bean = (Person) context.getBean("person");
报以下错误,原因:@Autowire注解默认是根据类型去匹配,但是此时有两个实现类,所以会出现错误,此时需要加上
@Qulifier注解告诉spring容器自动装配哪个名称的对象
Error creating bean with name 'person': Injection of autowired dependencies failed; nested exception is org.springframework.beans.factory.BeanCreationException: Could not autowire field: private com.dream.bean.Car com.dream.bean.Person.car; nested exception is org.springframework.beans.factory.NoUniqueBeanDefinitionException: No qualifying bean of type [com.dream.bean.Car] is defined: expected single matching bean but found 2: baoma,xianDai
at org.springframework.beans.factory.annotation.AutowiredAnnotationBeanPostProcessor.postProcessPropertyValues(AutowiredAnnotationBeanPostProcessor.java:334)
– @Authwired 注解也可以应用在数组类型的属性上, 此时 Spring 将会把所有匹配的 Bean 进行自动装配.
– @Authwired 注解也可以应用在集合属性上, 此时 Spring 读取该集合的类型信息, 然后自动装配所有与之兼容的 Bean.
– @Authwired 注解用在 java.util.Map 上时, 若该 Map 的键值为 String, 那么 Spring 将自动装配与之 Map 值类型兼容的 Bean, 此时 Bean 的名称作为键值
使用 @Resource 或 @Inject 自动装配 Bean
Resource不加名称默认也按照类型匹配,如果要匹配名称必须加入name属性
@Resource 是java的注释,但是Spring框架支持,@Resource指定注入哪个名称的对象
**@Resource(name="name")** == @Autowired + @Qualifier("name")
• Spring 还支持 @Resource 和 @Inject 注解,这两个注解和 @Autowired 注解的功用类似
• @Resource 注解要求提供一个 Bean 名称的属性,若该属性为空,则自动采用标注处的变量或方法名作为 Bean 的名称
• @Inject 和 @Autowired 注解一样也是按类型匹配注入的 Bean, 但没有 reqired 属性
8.1.4 初始化和销毁方法
初始化和销毁方法等同于配置文件添加的init-method和destroy-method功能,
例:Person类中init方法和destroy方法添加如**解:
第九章 Bean生命过程
• Spring IOC 容器可以管理 Bean 的生命周期, Spring 允许在 Bean 生命周期的特定点执行定制的任务.
• Spring IOC 容器对 Bean 的生命周期进行管理的过程:
– 通过构造器或工厂方法创建 Bean 实例
– 为 Bean 的属性设置值和对其他 Bean 的引用
– 调用 Bean的初始化方法**
– Bean 可以使用了
– 当容器关闭时,调用 Bean的销毁方法
• 在 Bean 的声明里设置 init-method 和 destroy-method 属性, 为 Bean 指定初始化和销毁方法, scope使用prototype的时候destroy-method方法对无效,@preDestroy注解也是一样
第十章 Spring整合JUnit测试
spring整合junit,为我们提供了方便的测试方式
1、导包:在spring-02-annotation项目中再加入如下包
spring-test-4.2.8.jar
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>4.3.8.RELEASE</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
2.创建测试类
//创建容器
@RunWith(SpringJUnit4ClassRunner.class)
// 指定创建容器时使用哪个配置文件
@ContextConfiguration("classpath:applicationContext.xml")
public class RunWithTest {
// 将名为user的对象注入到u变量中
@Autowired
private Person p;
@Test
public void testCreatePerson() {
System.out.println(p);
}
}